结构是c语言中比较重要的一部分,但是我的使用频率不高,为了防止遗忘,就写了这篇。不要说我能不用结构就不用结构,每个事物的存在都有其必然性,在你需要的时候你就会想起它啦!
如果有很多个结构,比较容易搞混,尤其是结构带着数组、指针,再写在函数里面就更热闹了。下面这个图写着我满脸的开心。🙂
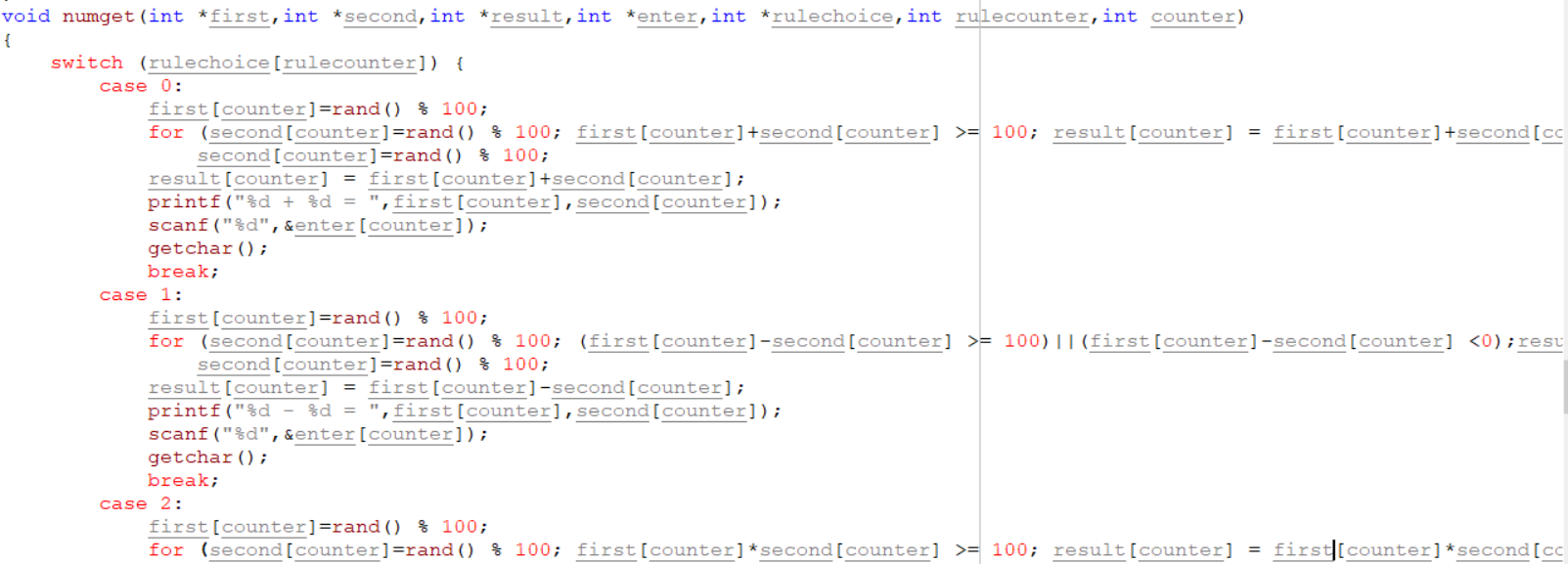
结构体的定义
1 2 3 4 5 6
| struct 结构体名{ 类型名1 成员名1; 类型名2 成员名2; …… 类型名n 成员名n; };
|
1 2 3 4 5
| struct Student{ char*name; int age; float height; };
|
结构体变量的定义(三种方法)
先定义结构体类型,再定义变量
1 2 3 4 5 6 7 8
| struct Student{ char*name; int age; float height; }; struct Student stu;
|
定义结构体类型的同时定义变量
1 2 3 4 5
| struct Student{ char*name; int age; float height; }stu;
|
直接定义结构体类型变量,省略类型名
1 2 3 4 5 6
| struct { char*name; int age; float height; }stu;
|
结构体的注意点
不允许对结构体本身递归定义,但是结构体内可以包含别的结构体。
定义结构体类型,只是说明了该类型的组成情况,并没有给它分配存储空间,就像系统不为int类型本身分配空间一样。只有当定义属于结构体类型的变量时,系统才会分配存储空间给该变量
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| #include <stdio.h> int main() { struct Date{ int year; int month; int day; }; struct Student{ char *name; struct Date birthday; }; struct Student stu; stu.name="someone"; stu.birthday.year=2018; stu.birthday.month=8; stu.birthday.day=15; printf("%s\n",stu.name); printf("%d %d %d\n",stu.birthday.year,stu.birthday.month,stu.birthday.day); }
|
结构体的初始化
将各成员的初值,按顺序地放在一对大括号{}中,并用逗号分隔,一一对应赋值。
注意一定是用大括号!!字符型的用””,int or float就用数值。
只能在定义变量的同时进行初始化赋值,初始化赋值和变量的定义不能分开。
1 2 3 4 5
| struct Student { char *name; int age; }; struct Student stu = {"MJ", 27};
|
1 2 3 4 5 6 7 8 9 10 11
| #include <stdio.h> int main() { struct { char *name; int age; }stu[2]={{"ta",19},{"ni",19}}; printf("%s %d\n",stu[0].name,stu[0].age); printf("%s %d\n",stu[1].name,stu[1].age); }
|
结构体的使用
一般对结构体变量的操作是以成员为单位进行的,引用的一般形式为:结构体变量名.成员名
(2)行对结构体的age成员进行了赋值。”.”称为成员运算符,它在所有运算符中优先级最高。
1 2 3 4 5 6
| struct Student { char *name; int age; }; struct Student stu; stu.age = 27;
|
相同类型的结构体变量之间可以进行整体赋值。
1 2 3 4 5 6 7 8 9
| 3、相同类型的结构体变量之间可以进行整体赋值 struct Student { char *name; int age; }; struct Student stu1 = {"MJ", 27}; struct Student stu2 = stu1; printf("age is %d", stu2.age);
|
结构体作为函数参数
我记得期末考试的时候好像出了这么一道题。
将结构体变量作为函数参数进行传递时,其实传递的是全部成员的值,也就是将实参中成员的值一一赋值给对应的形参成员。因此,形参的改变不会影响到实参。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| #include <stdio.h> struct Student{ int age; } ; void test(struct Student stu){ printf("修改前的形参:%d \n",stu.age); stu.age = 10; printf("修改后的形参: %d \n",stu.age); }
int main(){ struct Student stu = {30}; printf("修改前的实参:%d \n",stu.age); test(stu); printf("修改后的实参: %d \n",stu.age); return 0; }
|
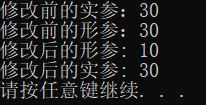
可以把结构作为函数参数,传参方式与其他类型的变量或指针类似。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| #include <stdio.h> #include <string.h> struct Books { char title[50]; char author[50]; char subject[100]; int book_id; };
void printBook( struct Books book ); int main( ) { struct Books Book1; struct Books Book2; strcpy( Book1.title, "C Programming"); strcpy( Book1.author, "Nuha Ali"); strcpy( Book1.subject, "C Programming Tutorial"); Book1.book_id = 6495407; strcpy( Book2.title, "Telecom Billing"); strcpy( Book2.author, "Zara Ali"); strcpy( Book2.subject, "Telecom Billing Tutorial"); Book2.book_id = 6495700; printBook( Book1 ); printBook( Book2 ); return 0; } void printBook( struct Books book ) { printf( "Book title : %s\n", book.title); printf( "Book author : %s\n", book.author); printf( "Book subject : %s\n", book.subject); printf( "Book book_id : %d\n", book.book_id); }
|
指向结构体的指针变量
1、每个结构体变量都有自己的存储空间和地址,因此指针也可以指向结构体变量
2、结构体指针变量的定义形式:struct 结构体名称 *指针变量名
3、有了指向结构体的指针,那么就有3种访问结构体成员的方式
(1)、结构体变量名.成员名
(2)、(*指针变量名).成员名
(3)、指针变量名->成员名
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| #include<stdio.h> int main() { struct Student{ char *name; int age; }; struct Student stu = {"OJ",18}; struct Student *p; p = &stu; printf("name=%s,age = %d \n",stu.name,stu.age); printf("name=%s,age = %d \n",(*p).name,(*p).age); printf("name=%s,age = %d \n",p->name,p->age); return 0; }
|
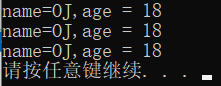
您可以定义指向结构的指针,方式与定义指向其他类型变量的指针相似,如下所示:
struct Books *struct_pointer;
现在,您可以在上述定义的指针变量中存储结构变量的地址。为了查找结构变量的地址,请把 & 运算符放在结构名称的前面,如下所示:
struct_pointer = &Book1;
为了使用指向该结构的指针访问结构的成员,您必须使用 -> 运算符,如下所示:
struct_pointer->title;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| #include <stdio.h> #include <string.h> struct Books { char title[50]; char author[50]; char subject[100]; int book_id; };
void printBook( struct Books *book ); int main( ) { struct Books Book1; struct Books Book2; strcpy( Book1.title, "C Programming"); strcpy( Book1.author, "Nuha Ali"); strcpy( Book1.subject, "C Programming Tutorial"); Book1.book_id = 6495407; strcpy( Book2.title, "Telecom Billing"); strcpy( Book2.author, "Zara Ali"); strcpy( Book2.subject, "Telecom Billing Tutorial"); Book2.book_id = 6495700; printBook( &Book1 ); printBook( &Book2 ); return 0; } void printBook( struct Books *book ) { printf( "Book title : %s\n", book->title); printf( "Book author : %s\n", book->author); printf( "Book subject : %s\n", book->subject); printf( "Book book_id : %d\n", book->book_id); }
|
参考文献
C 结构体| 菜鸟教程
C语言入门教程24-结构体· C语言编程基础· 看云
c语言中的关键字struct(结构体)的用法- 简书